Published
- 8 min read
Rotation Facts 1
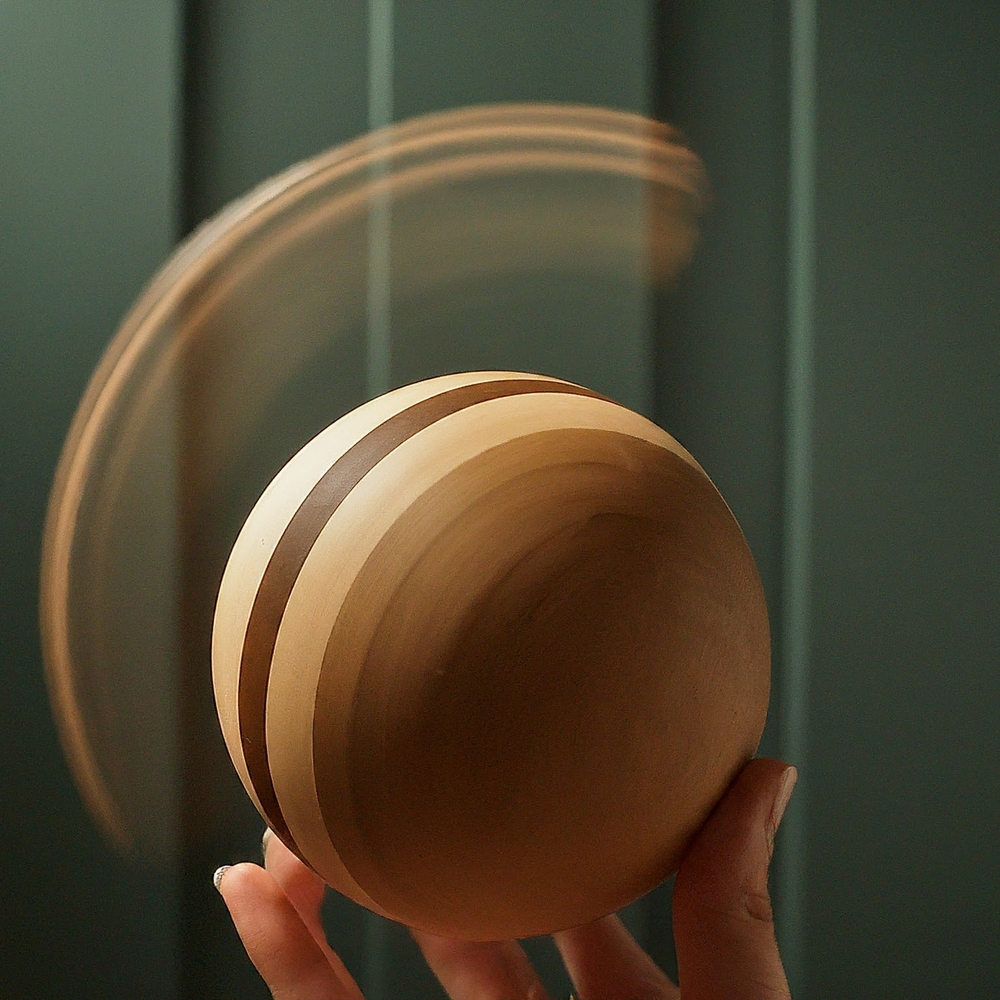
Rotation
- Rotation is the circular movement of an object around a fixed point or axis.
- It is a type of motion that involves the rotation of an object about its center of mass.
- The fixed point or axis around which the object rotates is called the axis of rotation.
- The object rotates in a circular path, and each point on the object moves in a circular trajectory.
- The angle through which the object rotates is measured in degrees or radians.
- The direction of rotation can be clockwise or counterclockwise.
- The speed of rotation is measured in terms of angular velocity, which is the rate of change of the angle with respect to time.
- The rotational motion of an object can be described using rotational kinematics, dynamics, and energy concepts.
- Rotational motion is governed by Newton’s laws of motion and the principles of conservation of angular momentum.
- Rotational motion is commonly observed in objects such as wheels, gears, planets, and celestial bodies.
function calculateAngularMomentum(momentOfInertia, angularVelocity) {
// L = Iω
return momentOfInertia * angularVelocity;
}
function calculateRotationalKineticEnergy(momentOfInertia, angularVelocity) {
// KE = 1/2 * I * ω^2
return 0.5 * momentOfInertia * Math.pow(angularVelocity, 2);
}
function calculateMomentOfInertia(mass, distance) {
// I = m*r^2
return mass * Math.pow(distance, 2);
}
function calculateTorque(force, radius, angleInDegrees) {
// T = F*r*sin(θ)
return force * radius * Math.sin(angleInDegrees * Math.PI / 180);
}
function calculateAngularVelocity(angleInRadians, timeInSeconds) {
// ω = Δθ / Δt
return angleInRadians / timeInSeconds;
}
function calculateAngularAcceleration(angularVelocity, timeInSeconds) {
// α = Δω / Δt
return angularVelocity / timeInSeconds;
}
Angular Momentum
- Angular momentum is a vector quantity that describes the rotational motion of an object.
- It is defined as the product of the moment of inertia and the angular velocity of the object.
- Angular momentum is a conserved quantity in the absence of external torques.
- The direction of angular momentum is perpendicular to the plane of rotation and follows the right-hand rule.
- Angular momentum plays a crucial role in understanding the stability and motion of rotating objects.
- The conservation of angular momentum is used to explain various phenomena in physics, such as the motion of planets and satellites.
- Angular momentum is an essential concept in classical mechanics, quantum mechanics, and celestial mechanics.
- The angular momentum of an object can change due to external torques acting on the object.
- The angular momentum of a system remains constant if the net external torque acting on the system is zero.
- Angular momentum is a fundamental quantity in the study of rotational dynamics and motion.
function calculateAngularMomentum(momentOfInertia, angularVelocity) {
// L = Iω
return momentOfInertia * angularVelocity;
}
function calculateAngularImpulse(torque, timeInSeconds) {
// L = τΔt
return torque * timeInSeconds;
}
function calculateAngularMomentumTorque(torque, timeInSeconds) {
// L = τΔt
return torque * timeInSeconds;
}
function calculateAngularMomentumForce(force, radius, angleInDegrees) {
// L = r x F
return radius * force * Math.sin(angleInDegrees * Math.PI / 180);
}
Torque
- Torque is a measure of the rotational force applied to an object.
- It is defined as the product of the force applied and the distance from the axis of rotation.
- Torque is a vector quantity that has both magnitude and direction.
- The direction of torque is perpendicular to the plane of rotation and follows the right-hand rule.
- Torque causes an object to rotate around an axis or pivot point.
- The magnitude of torque depends on the force applied, the distance from the axis of rotation, and the angle of application.
- Torque is measured in units of newton-meters (Nm) or foot-pounds (ft-lb).
- Torque is used to describe the rotational motion of objects and systems.
- Torque is essential in understanding the equilibrium, stability, and motion of rotating objects.
- Torque is a fundamental concept in physics, engineering, and mechanics.
function calculateTorque(force, radius, angleInDegrees) {
// T = F*r*sin(θ)
return force * radius * Math.sin(angleInDegrees * Math.PI / 180);
}
function calculateTorqueMomentOfInertia(angularAcceleration, momentOfInertia) {
// T = Iα
return momentOfInertia * angularAcceleration;
}
function calculateTorqueAngularMomentum(angularMomentum, timeInSeconds) {
// T = ΔL / Δt
return angularMomentum / timeInSeconds;
}
function calculateTorquePower(angularVelocity, torque) {
// P = τω
return torque * angularVelocity;
}
Moment of Inertia
- The moment of inertia is a measure of an object’s resistance to changes in its rotational motion.
- It depends on the mass distribution of the object and the axis of rotation.
- The moment of inertia is defined as the sum of the products of the mass of each particle in the object and the square of its distance from the axis of rotation.
- The moment of inertia is a scalar quantity that is always positive.
- The moment of inertia of an object depends on its shape, size, and mass distribution.
- The moment of inertia is used to calculate the rotational kinetic energy and angular momentum of an object.
- The moment of inertia of a system of particles is the sum of the moments of inertia of each particle.
- The moment of inertia is an essential concept in rotational dynamics and motion.
- The moment of inertia of an object can change if the mass distribution or axis of rotation changes.
- The moment of inertia is crucial in understanding the stability and motion of rotating objects.
function calculateMomentOfInertia(mass, distance) {
// I = m*r^2
return mass * Math.pow(distance, 2);
}
function calculateMomentOfInertiaRod(mass, length) {
// I = (1/3) * m * L^2
return (1 / 3) * mass * Math.pow(length, 2);
}
function calculateMomentOfInertiaDisk(mass, radius) {
// I = (1/2) * m * R^2
return (1 / 2) * mass * Math.pow(radius, 2);
}
function calculateMomentOfInertiaSphere(mass, radius) {
// I = (2/5) * m * R^2
return (2 / 5) * mass * Math.pow(radius, 2);
}
function calculateMomentOfInertiaCylinder(mass, radius, height) {
// I = (1/2) * m * (R^2 + H^2)
return (1 / 2) * mass * (Math.pow(radius, 2) + Math.pow(height, 2));
}
Rotational Kinetic Energy
- Rotational kinetic energy is the energy associated with the rotational motion of an object.
- It is defined as the sum of the kinetic energies of all the particles in the object due to their rotational motion.
- Rotational kinetic energy depends on the moment of inertia and the angular velocity of the object.
- The rotational kinetic energy of an object is given by the formula KE = 1/2 * I * ω^2.
- Rotational kinetic energy is a scalar quantity that is always positive.
- Rotational kinetic energy is used to describe the energy of rotating objects and systems.
- The rotational kinetic energy of an object can change due to changes in its angular velocity or moment of inertia.
- Rotational kinetic energy is an essential concept in understanding the motion and dynamics of rotating objects.
- The total kinetic energy of an object is the sum of its translational kinetic energy and rotational kinetic energy.
- Rotational kinetic energy is crucial in various fields of physics, engineering, and mechanics.
function calculateRotationalKineticEnergy(momentOfInertia, angularVelocity) {
// KE = 1/2 * I * ω^2
return 0.5 * momentOfInertia * Math.pow(angularVelocity, 2);
}
function calculateRotationalKineticEnergyDisk(mass, radius, angularVelocity) {
// KE = (1/2) * m * R^2 * ω^2
return (1 / 2) * mass * Math.pow(radius, 2) * Math.pow(angularVelocity, 2);
}
function calculateRotationalKineticEnergySphere(mass, radius, angularVelocity) {
// KE = (2/5) * m * R^2 * ω^2
return (2 / 5) * mass * Math.pow(radius, 2) * Math.pow(angularVelocity, 2);
}
function calculateRotationalKineticEnergyCylinder(mass, radius, height, angularVelocity) {
// KE = (1/2) * m * (R^2 + H^2) * ω^2
return (1 / 2) * mass * (Math.pow(radius, 2) + Math.pow(height, 2)) * Math.pow(angularVelocity, 2);
}
function calculateRotationalKineticEnergyRod(mass, length, angularVelocity) {
// KE = (1/3) * m * L^2 * ω^2
return (1 / 3) * mass * Math.pow(length, 2) * Math.pow(angularVelocity, 2);
}
Angular Velocity
- Angular velocity is a vector quantity that describes the rate of change of the angle of rotation of an object.
- It is defined as the change in the angle of rotation with respect to time.
- Angular velocity is a measure of how fast an object is rotating around an axis.
- The SI unit of angular velocity is radians per second (rad/s).
- Angular velocity is a vector quantity that has both magnitude and direction.
- The direction of angular velocity is perpendicular to the plane of rotation and follows the right-hand rule.
- Angular velocity is used to describe the rotational motion of objects and systems.
- Angular velocity is related to the linear velocity of an object through the formula v = rω, where v is the linear velocity, r is the radius, and ω is the angular velocity
- Angular velocity plays a crucial role in understanding the dynamics and motion of rotating objects.
- Angular velocity is an essential concept in physics, engineering, and mechanics.
function calculateAngularVelocity(angleInRadians, timeInSeconds) {
// ω = Δθ / Δt
return angleInRadians / timeInSeconds;
}
function calculateAngularVelocityLinearVelocity(linearVelocity, radius) {
// ω = v / r
return linearVelocity / radius;
}
function calculateAngularVelocityFrequency(frequency) {
// ω = 2πf
return 2 * Math.PI * frequency;
}
function calculateAngularVelocityPeriod(period) {
// ω = 2π / T
return 2 * Math.PI / period;
}
function calculateAngularVelocityTangentialVelocity(tangentialVelocity, radius) {
// ω = v / r
return tangentialVelocity / radius;
}